Index notation
|
Read other articles:

Jonathan MajorsMajors pada 2018Lahir7 September 1989 (umur 34)Lompoc, California, USAPendidikanUniversity of North Carolina School of the Arts (Sarjana)Universitas Yale (Magistrat)PekerjaanPemeranTahun aktif2017 - sekarangTinggi183 cm (6 ft 0 in)Anak1 Jonathan Majors (lahir 7 September 1989)[1][2] adalah seorang pemeran asal Amerika Serikat. Ia meraih ketenaran usai membintangi film fitur independen The Last Black Man in San Francisco (2019). Pada 2020, i…
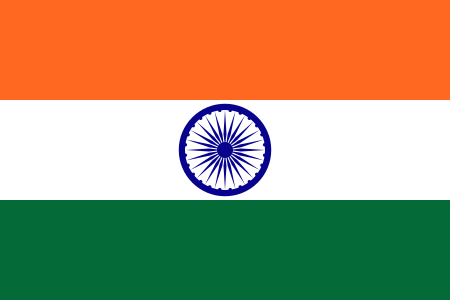
Dixit memfilmkan Dance Deewane pada 2018 Madhuri Dixit adalah seorang pemeran India yang dikenal atas karyanya dalam perfilman Bollywood. Ia memulai debutnya dengan berperan sebagai seorang mempelai muda dalam film 1984 Abodh.[1] Dixit tampil dalam beberapa film sepanjang dua tahun berikutnya, meliputi film drama Awara Baap (1985) dan Swati (1986), walaupun tak ada yang meraih sambutan besar.[1] Peran terobosannya datang pada 1988 dengan film cerita seru garapan N. Chandra Tezaab…

Koningsberger Jacob Christiaan Koningsberger (Hazerswoude, 17 Januari 1867 - 's-Gravenhage, 19 Maret 1951) adalah seorang dosen dan politikus berkebangsaan Belanda. Koningsberger merupakan Menteri Jajahan Belanda di kabinet sementara De Geer. Segera setelah lulus ia pindah ke Hindia Belanda dan di sana menduduki jabatan yang berbeda-beda di bidang ilmu pengetahuan. Di Buitenzorg (sekarang Kota Bogor) ia menjabat sebagai Direktur Kebun Raya Bogor antara tahun 1911-1917. Selepasnya dari jabatan di…

Yana's FriendsSutradaraArik KaplunProduserAnat BikelMoshe LevinsonMarek RozenbaumUri SabagDitulis olehArik KaplunSimon VinokurPemeranEvelyn KaplunNir LevyShmil Ben AriMosko AlkalaiDalia FriedlandPenata musikAvi BenjaminSinematograferValentin BelonogovPenyuntingEinat Glaser-ZarhinDistributorFriends of Film (A.S.)Tanggal rilis4 November 1999 (Israel)Durasi90 menitNegaraIsraelBahasaIbraniRusia Yana's Friends ([החברים של יאנה, HaHaverim shel Yana] Error: {{Lang-xx}}: text has itali…

Departemen Perencanaan New South Wales (bahasa Inggris: New South Wales Department of Planning, disingkat DOP) adalah sebuah departemen Pemerintah New South Wales dengan tugas administrasi sistem tata negara bagian dan penetapan sejumlah penerapan pembangunan. Departemen ini juga bertugas mengatur pelepasan tanah untuk perumahan baru tunduknya pemerintah lokal pada hukum tata negara bagian dalam penerapan pembangunan dan penzonaan. Kekuasaan Departemen sesuai dengan NSW Environmental Planning an…

3rd episode of the 2nd season of Seinfeld The JacketSeinfeld episodeEpisode no.Season 2Episode 3Directed byTom CheronesWritten byLarry David and Jerry SeinfeldProduction code205Original air dateFebruary 6, 1991 (1991-02-06)Guest appearances Lawrence Tierney as Alton Benes Frantz Turner as Salesman Suanne Spoke as Customer Harry Hart-Browne as Manager Episode chronology ← PreviousThe Pony Remark Next →The Phone Message Seinfeld season 2List of episodes The Jacket…
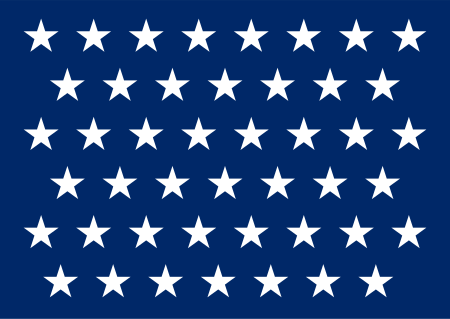
Pertempuran Teluk ManilaBagian dari Perang Spanyol-AmerikaCetak berwarna kontemporer, menunjukkan USS Olympia di latar depan kiri, memimpin Skuadron Asiatik Amerika Serikat melawan armada kapal Spanyol dekat Cavite. Sebuah potret sketsa dari Laksamana George Dewey ditampilkan di bagian kiri bawah.Tanggal1 Mei 1898LokasiDekat Manila, FilipinaHasil Kemenangan mutlak Amerika SerikatPihak terlibat Amerika Serikat Kerajaan SpanyolTokoh dan pemimpin George Dewey Patricio MontojoKekuatan Kek…

العلاقات الإستونية الصربية إستونيا صربيا إستونيا صربيا تعديل مصدري - تعديل العلاقات الإستونية الصربية هي العلاقات الثنائية التي تجمع بين إستونيا وصربيا.[1][2][3][4][5] مقارنة بين البلدين هذه مقارنة عامة ومرجعية للدولتين: وجه المقارنة إستون…

Ongoing COVID-19 viral pandemic in Wyoming, United States This article needs to be updated. Please help update this article to reflect recent events or newly available information. (September 2021) COVID-19 pandemic in WyomingDiseaseCOVID-19Virus strainSARS-CoV-2LocationWyoming, U.S.First outbreakCalifornia, U.S.Index caseSheridan CountyArrival dateMarch 11, 2020Confirmed cases196,100[1]Deaths2,125[1]Government websitecovid19.wyo.govPart of a series on theCOVID-19 pandemicScienti…

Число китайцев в Казахстане менялось с течением времени. В XIX и начале XX веков имели место различные миграции этнических меньшинств из Китая (англ.) (рус. в Казахстан, например, дунгане (хуэй), бежавшие от вооружённых сил династии Цин после неудавшегося восстания 1862-1…

العلاقات الإسواتينية الغرينادية إسواتيني غرينادا إسواتيني غرينادا تعديل مصدري - تعديل العلاقات الإسواتينية الغرينادية هي العلاقات الثنائية التي تجمع بين إسواتيني وغرينادا.[1][2][3][4][5] مقارنة بين البلدين هذه مقارنة عامة ومرجعية للدولتي…

У этого термина существуют и другие значения, см. Истребитель танков (значения). Советское лёгкое самоходное орудие СУ-76.Боевая машина 2П26 в Военно-историческом музее артиллерии, инженерных войск и войск связи.Польские танкисты на учениях, ПТУРСы на 2П27 или «Шмеле».ИТ-1 в экс…

Type of marketing strategy A billboard for Sanford Health placed on the exterior of the Target Center, adjacent to Target Field, so that it is visible from within to compete with a sponsorship held by a competitor. Ambush marketing or ambush advertising is a marketing strategy in which an advertiser ambushes an event to compete for exposure against other advertisers. The term was coined by marketing strategist Jerry Welsh, while he was working as the manager of global marketing efforts for Ameri…

هذه المقالة يتيمة إذ تصل إليها مقالات أخرى قليلة جدًا. فضلًا، ساعد بإضافة وصلة إليها في مقالات متعلقة بها. (مارس 2019) بيترو لونجو (بالإيطالية: Pietro Longo) معلومات شخصية الميلاد 29 أكتوبر 1935 (89 سنة) روما مواطنة إيطاليا عضو في البروباغاندا الثانية [لغات أخرى]̴…
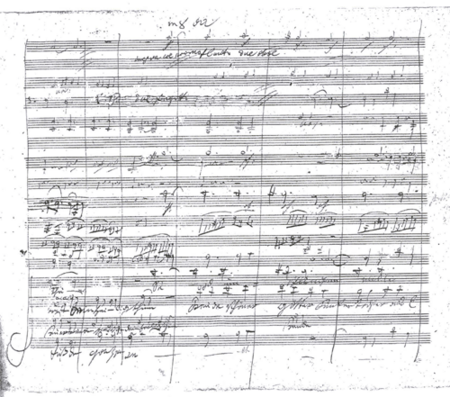
1974–1979 national anthem of Rhodesia Rise, O Voices of RhodesiaA page from Beethoven's original manuscriptFormer national anthem of Rhodesia and Zimbabwe-RhodesiaAlso known asVoices of Rhodesia[1]LyricsMary Bloom, 1974[1]MusicLudwig van Beethoven, 1824[2] (arranged by Ken MacDonald, 1974)AdoptedAugust 1974 (1974-08)[1]RelinquishedDecember 1979 (1979-12)Preceded byGod Save the QueenSucceeded byIshe Komborera Africa (As national anthem of Zimb…

Borough in Estonia Place in Lääne County, EstoniaTaeblaUus Street in TaeblaTaeblaCoordinates: 58°57′2″N 23°43′31″E / 58.95056°N 23.72528°E / 58.95056; 23.72528Country EstoniaCounty Lääne CountyParish Lääne-Nigula ParishPopulation (01.01.2010) • Total929 Taebla is a small borough (alevik) in Lääne County, Estonia,[1] the administrative centre of Lääne-Nigula Parish. It has a population of 929 (as of 1 January 2010).[2]…

American pizzeria chain Patxi's Chicago PizzaIndustryDiningFounded2004; 20 years ago (2004)FounderWilliam Freeman and Francisco “Patxi” AzpirozHeadquartersSausalito, California, USAProductsPizzaParentKarpReilly LLCWebsitehttps://www.patxispizza.com/ Patxi's (pronounced pah-cheese)[1] is a small pizzeria chain based in Sausalito, California.[2] History Patxi's was founded by William Freeman and Francisco “Patxi” Azpiroz.[3] The restaurant chain sp…

Villeneuve-le-Roi Photo panoramique de la façade de la mairie. Blason Logo Administration Pays France Région Île-de-France Département Val-de-Marne Arrondissement L'Haÿ-les-Roses Intercommunalité Métropole du Grand Paris Maire Mandat Didier Gonzales 2020-2026 Code postal 94290 Code commune 94077 Démographie Gentilé Villeneuvois Populationmunicipale 21 129 hab. (2021 ) Densité 2 515 hab./km2 Géographie Coordonnées 48° 44′ 00″ nord, 2° 25…

See also: List of historic places in Greater Vancouver Regional District, List of historic places in Greater Vancouver North Shore, List of historic places in Surrey, and List of historic places in Vancouver Map all coordinates using OpenStreetMap Download coordinates as: KML GPX (all coordinates) GPX (primary coordinates) GPX (secondary coordinates) The following list includes all of the Canadian Register of Historic Places listings in New Westminster, British Columbia. Name Address Coordinates…

This article needs additional citations for verification. Please help improve this article by adding citations to reliable sources. Unsourced material may be challenged and removed.Find sources: Rugby union in England – news · newspapers · books · scholar · JSTOR (July 2012) (Learn how and when to remove this message) Rugby union in EnglandCelebrations in Trafalgar Square after England won the 2003 Rugby World Cup.CountryEnglandGoverning bodyRugby Footbal…